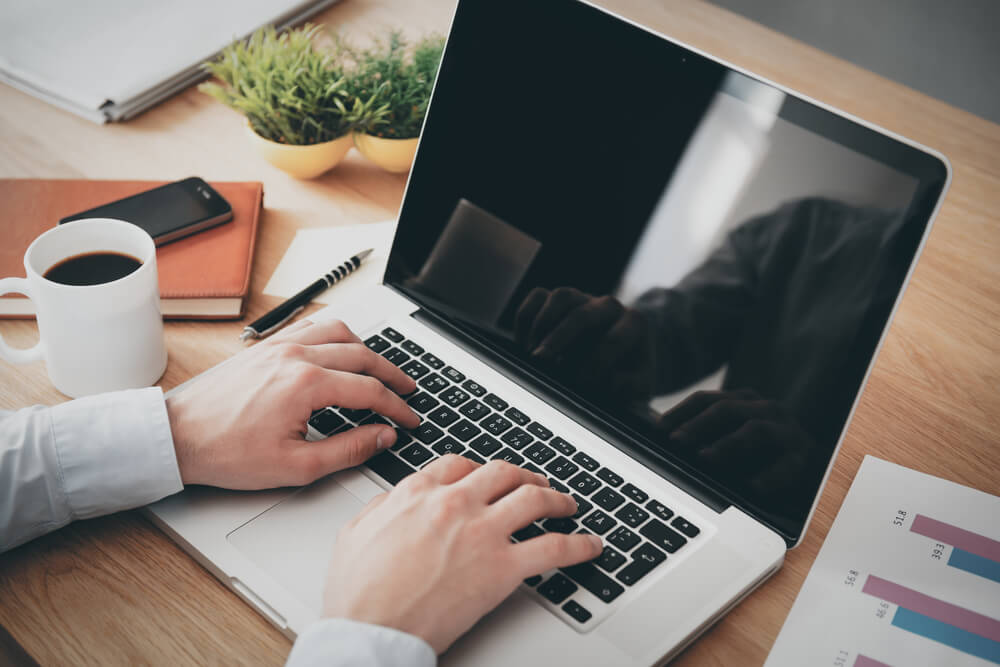
اینجا سرآغاز تولد یک مهندس ماهر است
هیلتون، برگزارکننده دورههای آموزشی آنلاین برنامه نویسی و طراحی وب و علم داده، با ۱۳ سال سابقه، از شروع یادگیری تا استخدام در بازار کار در کنار دانشجو است.
مشاهده دورههاتازهترین آموزش ها
آموزش هایی که به تازگی منتشر شدهاند
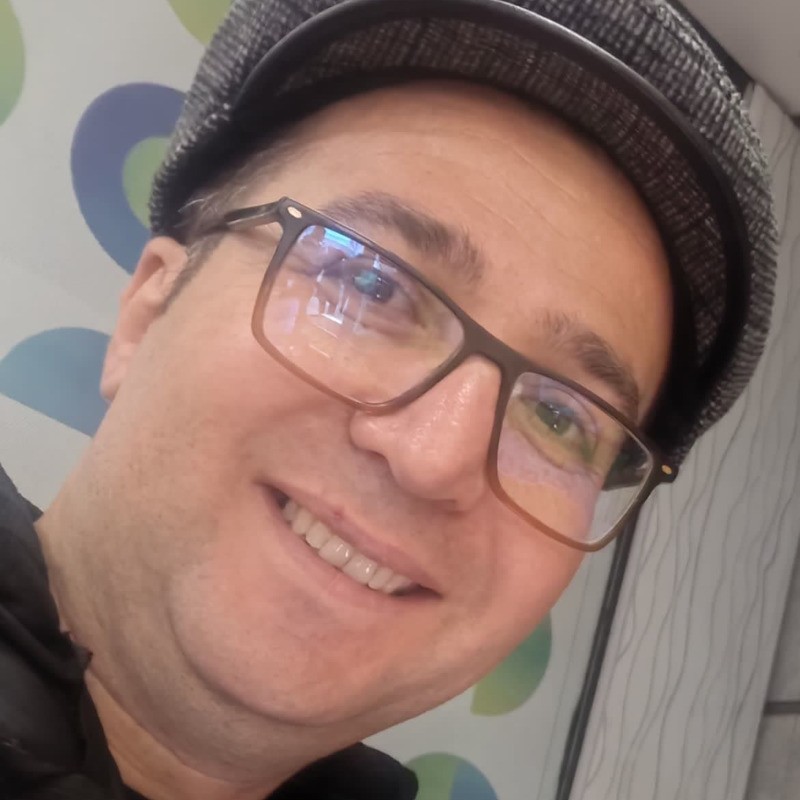
دروه Asp.net MVC Core جهت ورود به بازار کار
-
1 درس
-
15 ساعت
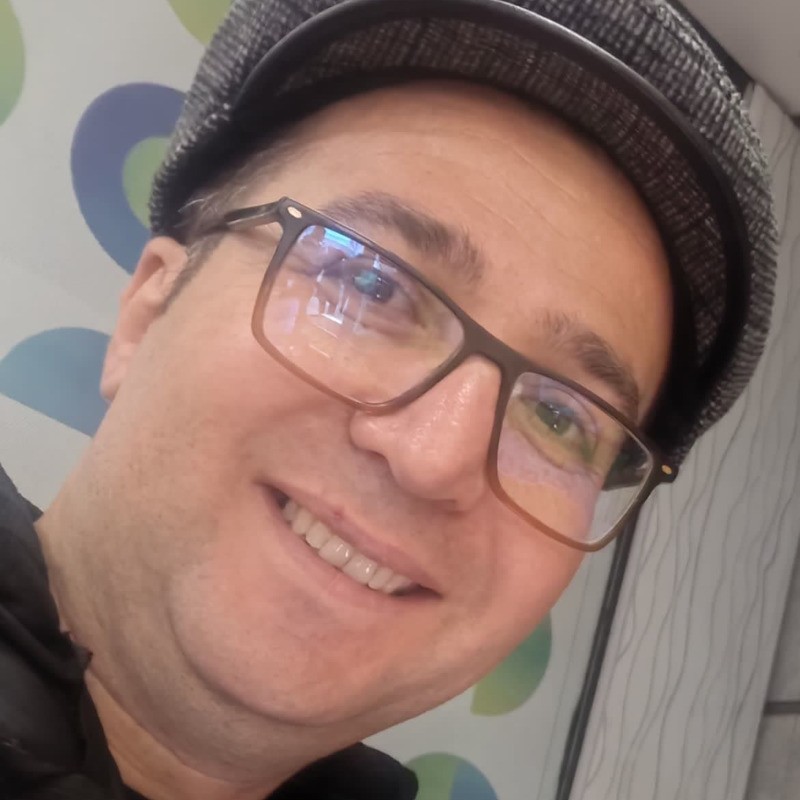
آموزش جاوا اسکریپت JavaScript+ریفکتور کد و کلین کد
-
1 درس
-
7.30ساعت
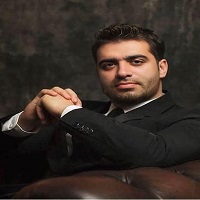
آموزش پروژه محور طراحی سایت فروشگاهی دیجی کالا (Digikala ) با زبان Asp.Net MVC تحت سی شارپ (#C)
-
1 درس
-
53 ساعت
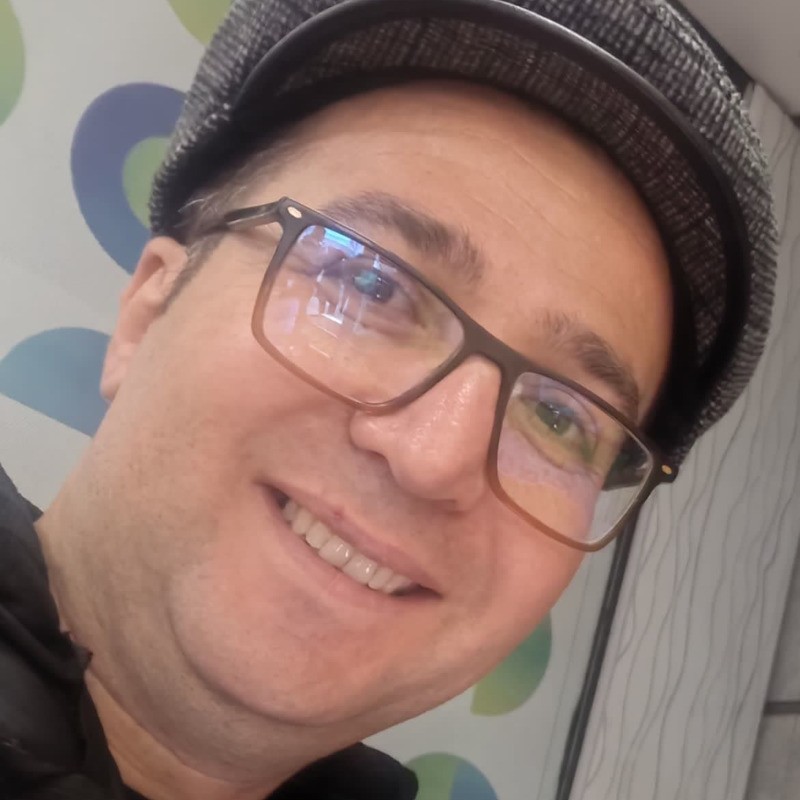
دروه Asp.net MVC Core جهت ورود به بازار کار
-
1 درس
-
15 ساعت
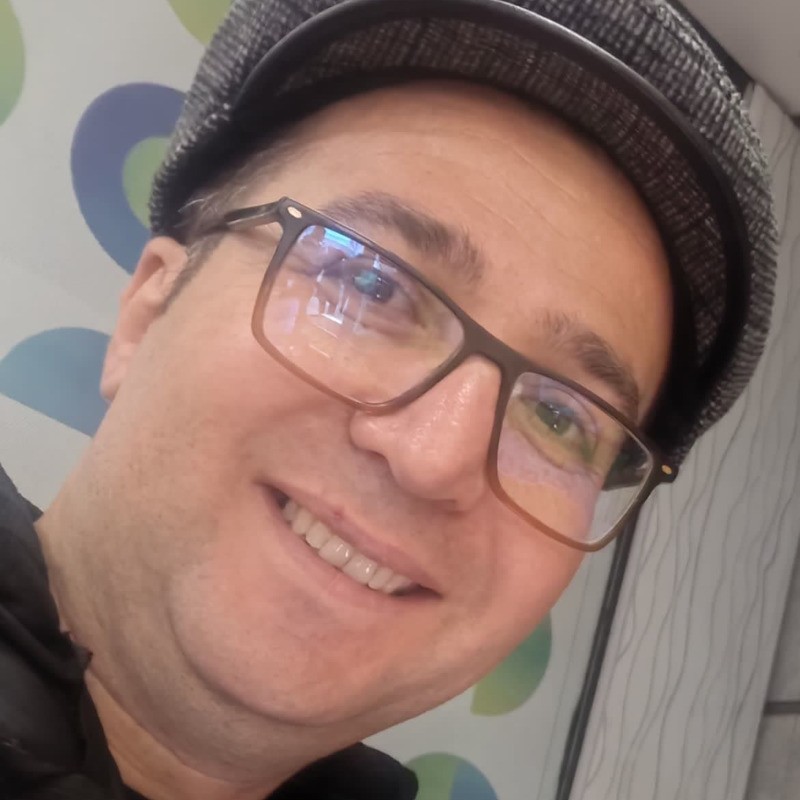
آموزش جاوا اسکریپت JavaScript+ریفکتور کد و کلین کد
-
1 درس
-
7.30ساعت
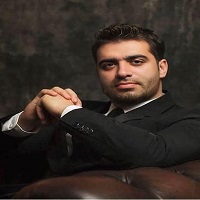
آموزش پروژه محور طراحی سایت فروشگاهی دیجی کالا (Digikala ) با زبان Asp.Net MVC تحت سی شارپ (#C)
-
1 درس
-
53 ساعت
انتخاب کن و یاد بگیر!
تو میتونی! فقط کافیه قدرت شروع ناقص رو باور کنی...
مدرسهای برتر
گلچینی از برترین اساتید توسعهی نرم افزار ایران